sendEvent
The events method to send data through BaseHub. Flexible, type-safe and scoped by block.
import { sendEvent } from 'basehub/events'
Parameters
Example
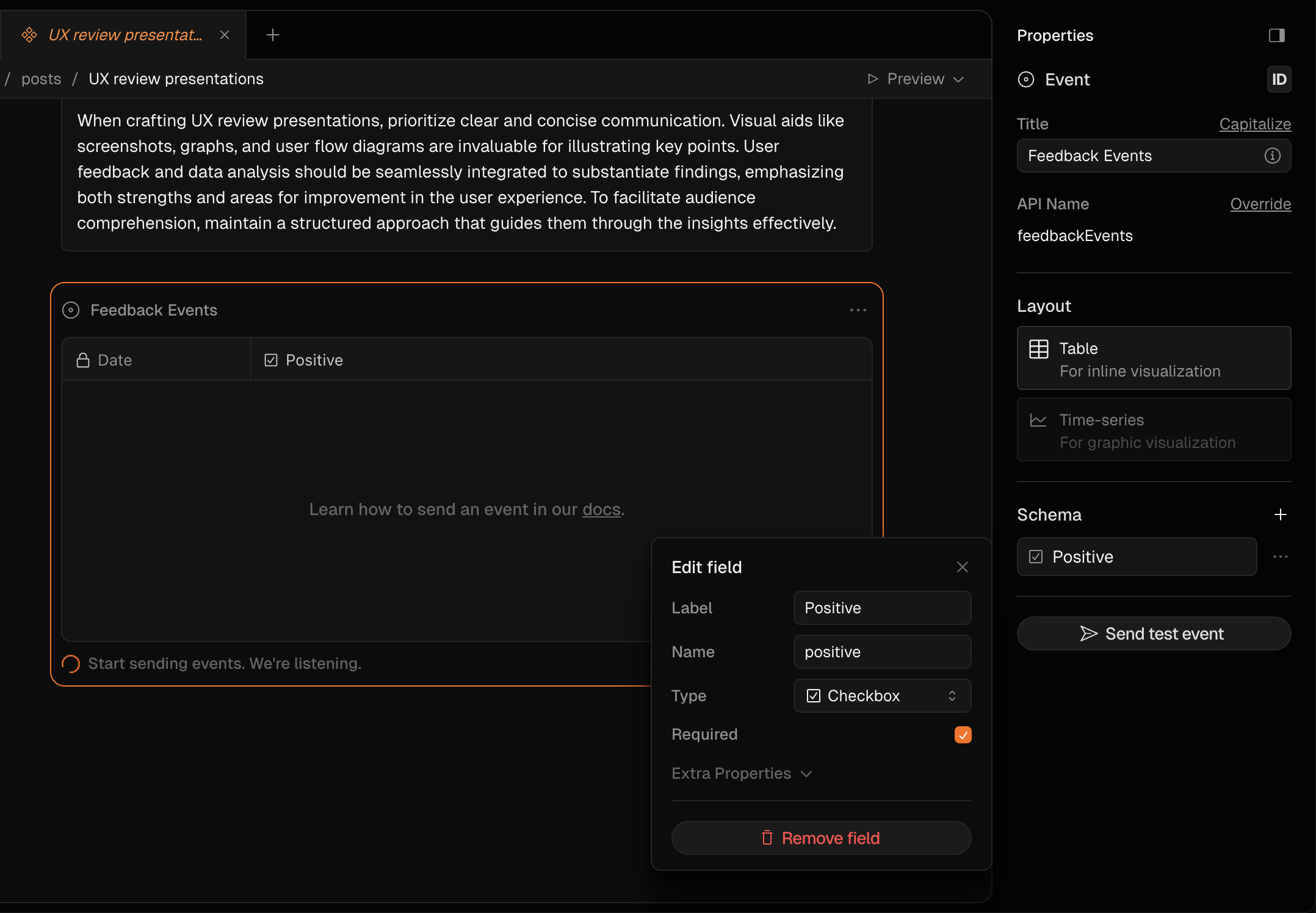
'use client'
// you'll need to run basehub before importing this type 👇🏼
import type { FeedbackEvents['ingestKey'] } from '~/.basehub/schema'
import { sendEvent } from 'basehub/events'
import { Card, IconButton } from '@radix-ui/themes'
import { ThumbsDown, ThumbsUp } from 'lucide-react'
import * as React from 'react'
export const Feedback = ({
ingestKey,
}: {
ingestKey: FeedbackEvents['ingestKey']
}) => {
const [sentFeedback, setSentFeedback] = React.useState<
'positive' | 'negative' | null
>(null)
const handleFeedback = (type: 'positive' | 'negative') => {
if (sentFeedback === type) return
sendEvent(ingestKey, { positive: type === 'positive' })
setSentFeedback(type)
}
return (
<Card variant="classic" size="3">
<IconButton onClick={() => handleFeedback('negative')}>
<ThumbsDown fill={sentFeedback === 'negative' ? 'var(--accent-12)' : 'none'} />
</IconButton>
<IconButton onClick={() => handleFeedback('positive')}>
<ThumbsUp fill={sentFeedback === 'positive' ? 'var(--accent-12)' : 'none'} />
</IconButton>
</Card>
)
}
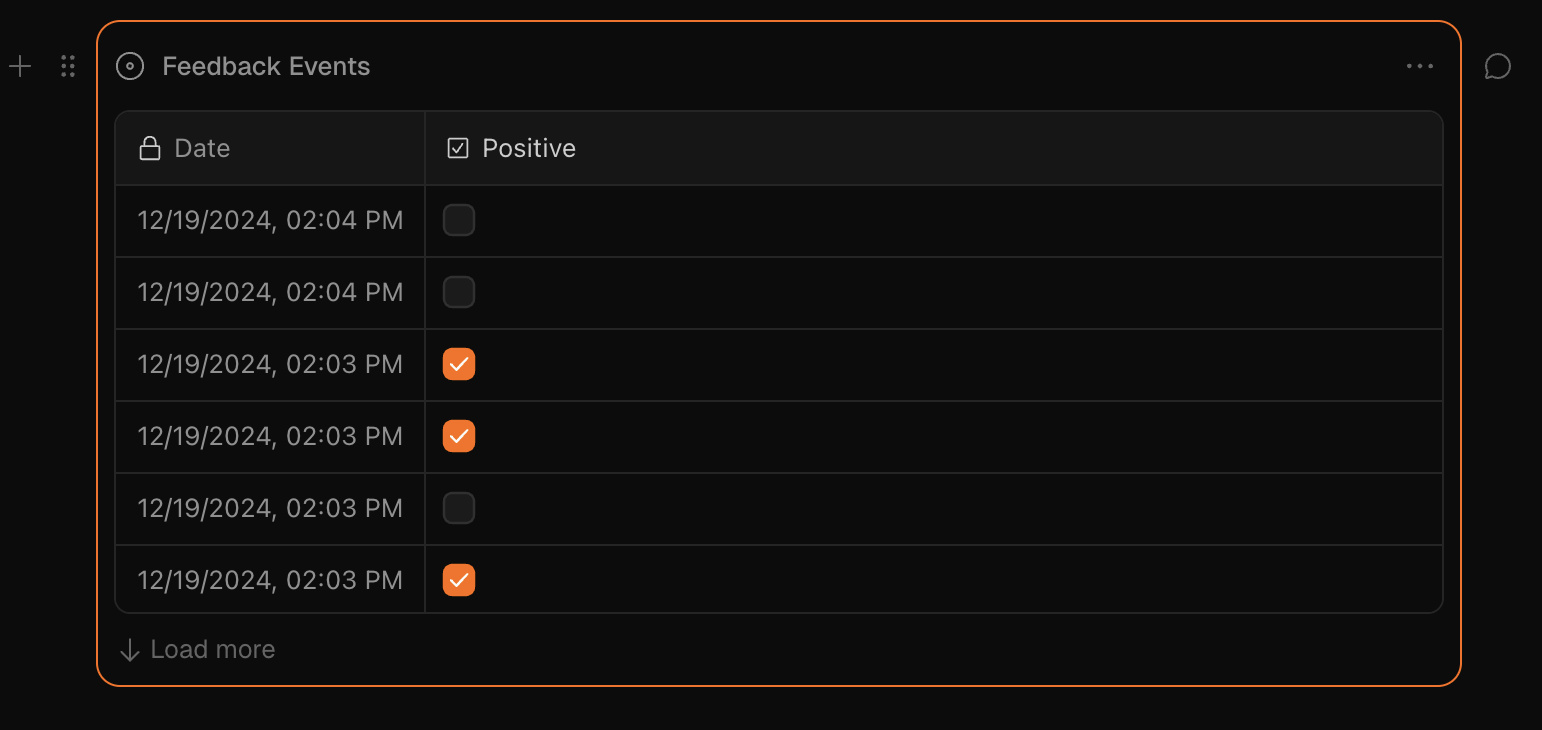